Joel and David chat about the big ideas and the essential questions of State Management and State Machines. Additionally, David also gives a walkthrough of XState, XState Visualizer, and the upcoming dev tools for XState.
Key Terms
XState - is a library for creating, interpreting, and executing finite state machines and statecharts, as well as managing invocations of those machines as actors.
State Machines - a mathematical model of computation. It is an abstract machine that can be in exactly one of a finite number of states at any given time.
Combinatorial Explosion - is the rapid growth of the complexity of a problem.
Resources
Visuals Explanation
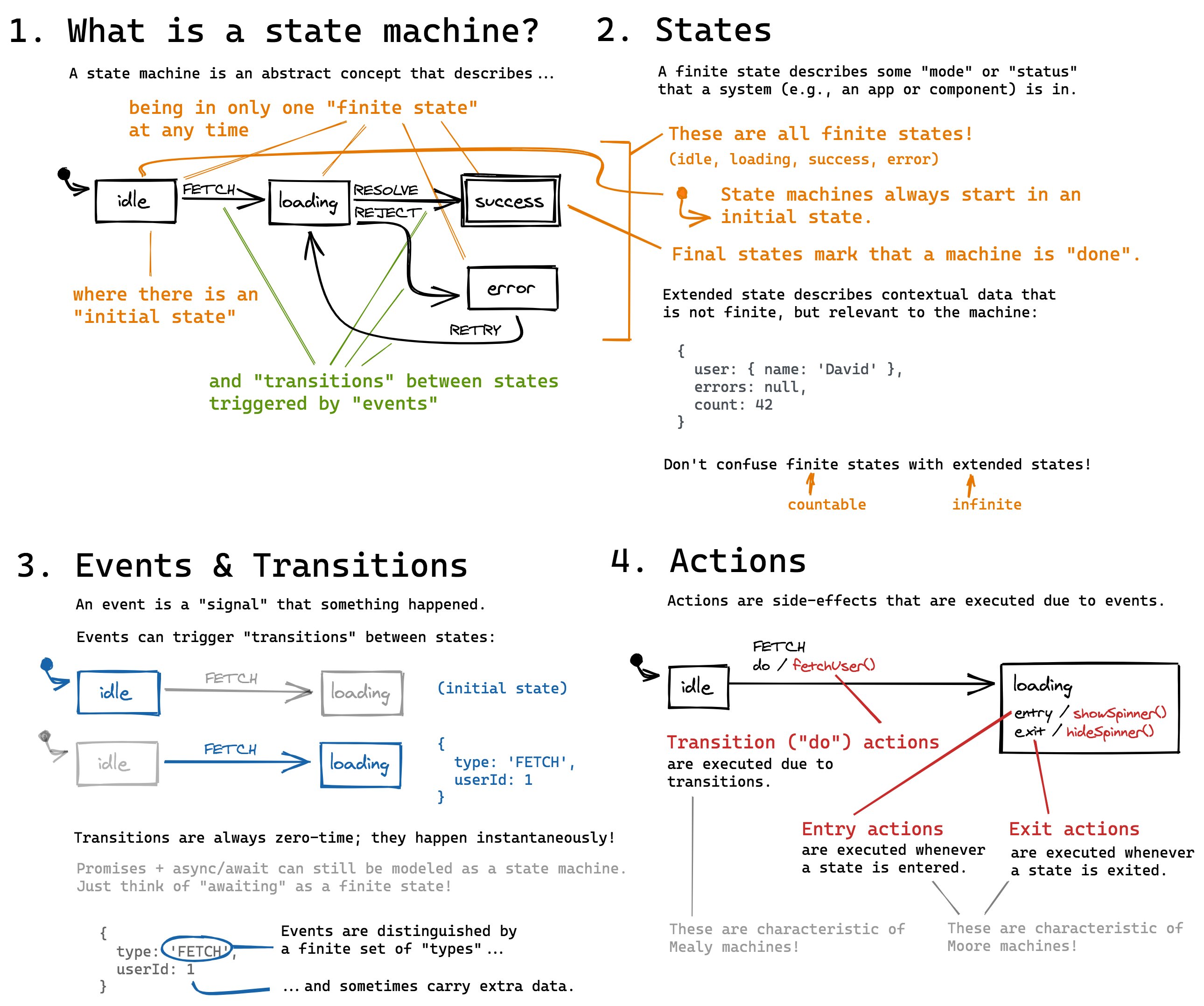
Source: https://www.stately.dev
These notes include: highlights, expansion of key terms, code blocks, and links to additional resources. They follow the same structure as the recording.
What are the big ideas of State Management?
- It all comes down to applying big ideas from the past, for example, State Machines.
- One of the biggest problems related to State Machines is performance.
- The problems with States are not new.
Why is this hard to understand these problems?
There's a mathematical explication to this. Once you have more than one piece of State in different places, you faced Combinatorial Explosion.
Combinatorial Explosion
A combinatorial explosion is the rapid growth of the complexity of a problem due to how the combinatorics of the problem is affected by the input, constraints, and bounds of the problem.
Combinatorics is an area of mathematics primarily concerned with counting, both as a means and an end in obtaining results.
An example of this is having ten main ingredients and having the output be 100 different dishes.
The most neglected variable is time when it comes to managing State. We can only think about this in abstract ways.
"We (developer) do most of the State management in our heads."
- There's no concrete way to communicate our understanding of States. This is one of the reasons why we have State Machines.
What are the Essential Questions when people work with State Management?
- First, identify all the pieces of State that you're dealing with.
- How is this information organized?
- How can this data change over time?
- How can you model this data?
What's a Finite State Machines?
- "XState is like Redux with Rules"
- A finite state machine is a mathematical model of computation that describes the behavior of a system that can be in only one state at any given time.
- Finite State, you can only be in one State at a time.
- It's more of a mode, "I'm awake & I'm asleep."
- It also describes how one can move from one State to another.
What's the difference between a State Machine and Statecharts?
- Finite State Machines can represent everything.
- Statecharts are a formalism for modeling stateful, reactive systems.
- David Harel presented this formalism as an extension to state machines in his 1987 paper Statecharts: A Visual Formalism for Complex Systems.
- A way to organize your states. "Sub-States."
- More resources:
How are State Machine addressing the big ideas of State management?
- Event-Action approach doesn't always make sense. A State Machine has a specific way of transitioning.
- A state transition defines what the next state is, given the current state and event. State transitions are defined on state nodes.
- Communication with humans and machines.
- More resources:
- Combinatorial Explosion
- State Machines is not something new; it has been implemented across different industries.
The Overlap of music and State Machines and Statecharts
- When you look at music, there are infinite ways to express a melody; inherently, there are rules.
- Music makes you a better visual thinker.
- This is an example when it comes to modeling State solutions.
- Music is centuries old; its theory is old. Computer science is the music theory of programming.
- Music theory answers why "this" works, much like when you start using a library, and you realized that it's an elegant solution to a specific problem.
Building a Statecharts for JavaScript
- The inspiration came from David's first job working with repetitive workflows. Wanting to solve this problem led to researching State Machines.
- Early days it was called "Estado," the Spanish word for State.
Demo of XState
Code sandbox demo
- Normal React Development:
<div className="App">
<h1>{toggled ? "Toggled" : "Nope"}</h1>
<h2>
{state.value} ({state.context.count})
</h2>
<button
onClick={() => {
send("TOGGLE");
}}
>
Toggle
</button>
</div>
...
- The problem is the all the business logic is inside the event handle.
- If you have more business requirements, you'll need to add 'defense logic' to handle more use cases.
Adding XState
import { createMachine, assign } from "xstate";
import { useMachine } from "@xstate/react";
const toggleMachine = createMachine({
initial: "untoggled",
context: {
count: 0
},
states: {
untoggled: {
on: { TOGGLE: "toggled" }
},
toggled: {
entry: assign({
count: context => context.count + 1
}),
on: {
TOGGLE: "untoggled"
},
after: {
1000: "untoggled"
}
}
}
});
useMachine(machine, options?)
- a React hook that interprets the given machine and starts a service that runs for the component's lifetime.
const [state, send] = useMachine(toggleMachine, { devTools: true });
console.log(state);
const [toggled, setToggled] = useState(false);
- We are creating an event-driven approach to handling toggles.
- All the logic is handle inside
toggleMachine
- With State Machines everything is an event (time is an event).
- Existing machines can be configured by passing the machine options as the 2nd argument of
useMachine(machine, options).
context: {
count: 0
},
- We can add a side-effects
count: context => context.count + 1
without affecting our main State.
XState Visualizer
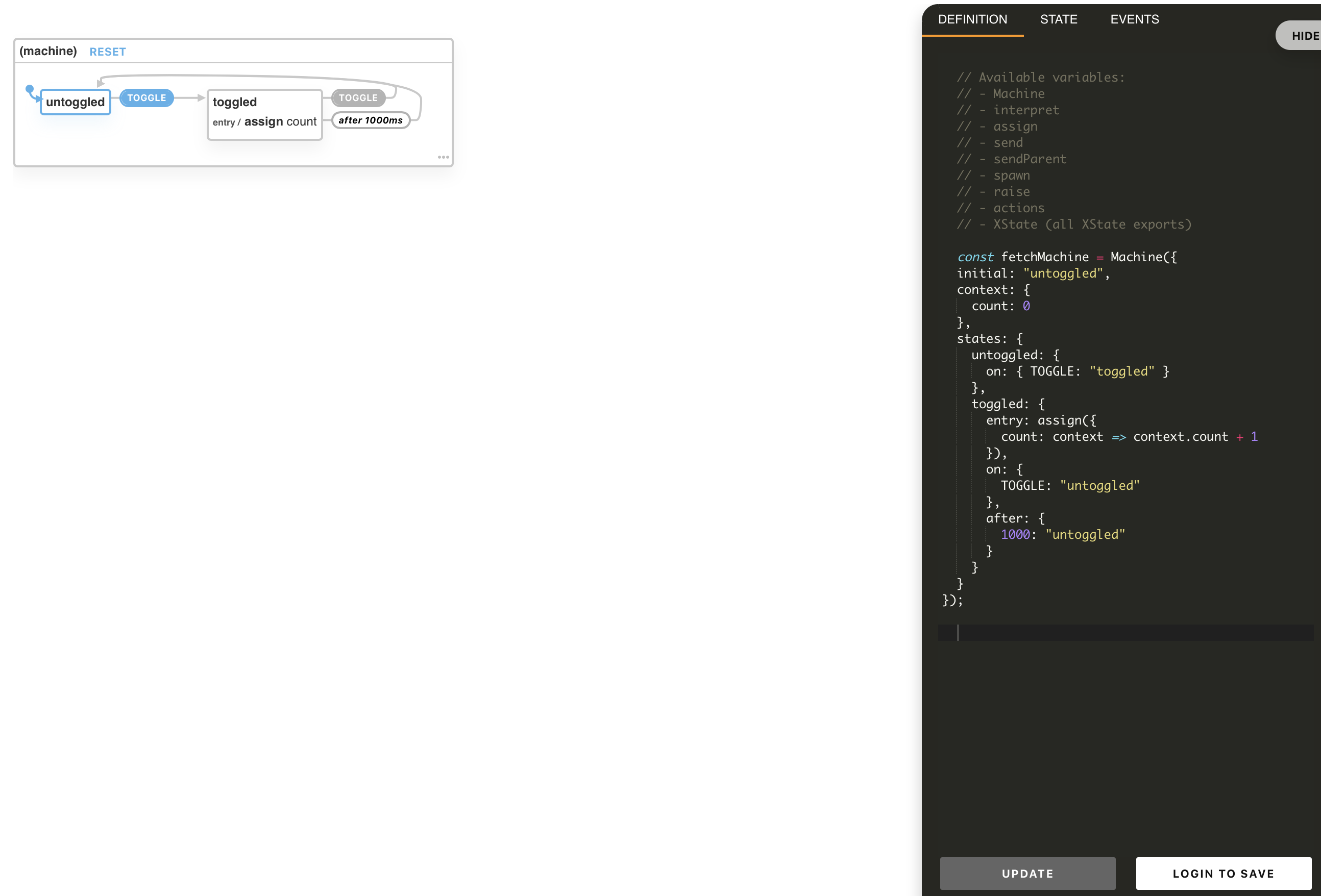
What's the workflow for design States?
- Pen and paper work pretty well. Modeling the behavior of a user is complicated.
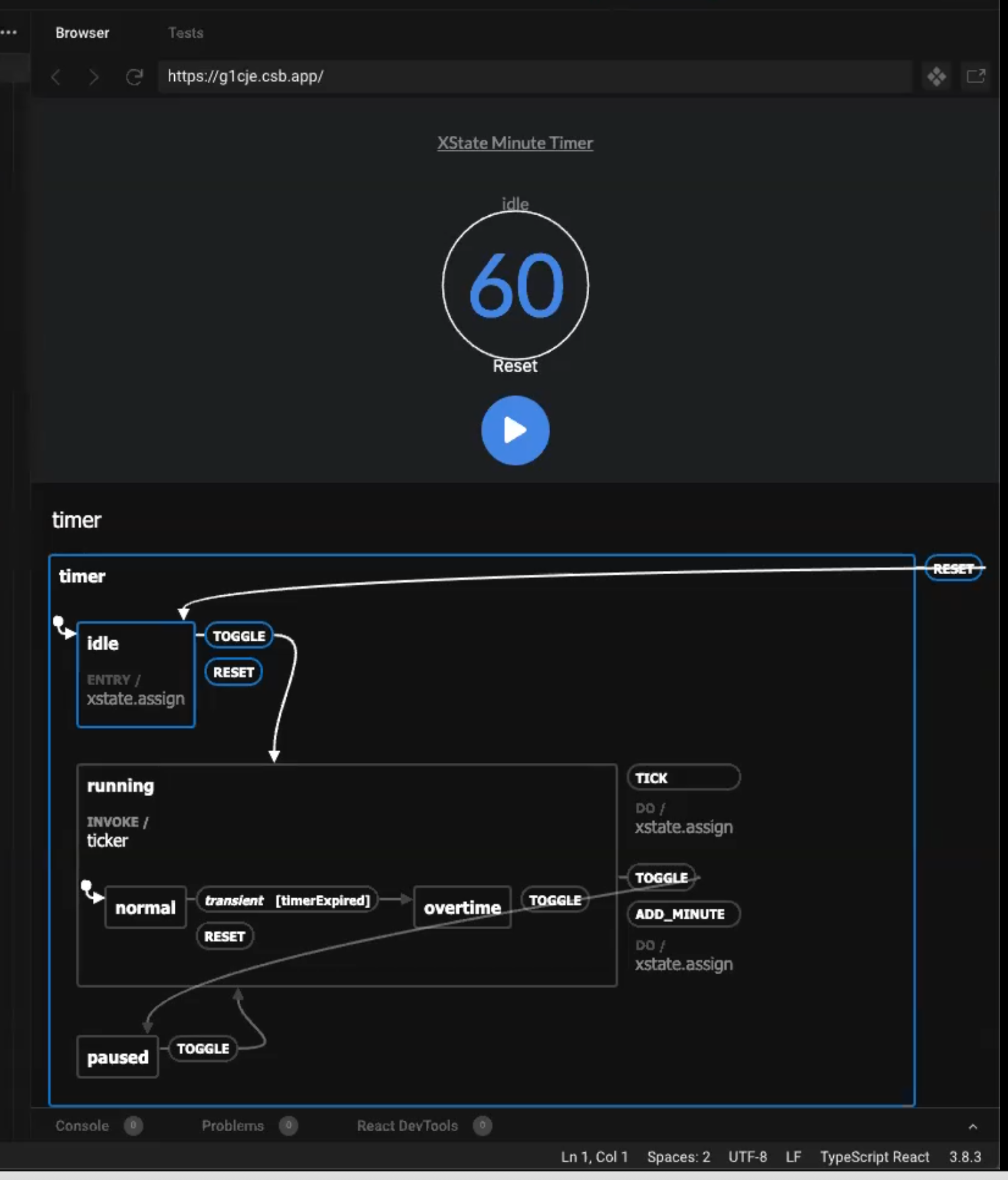
- Dev Tools for XState (coming soon)
- CodeSandbox also works pretty well for prototyping state.
When is XState not appropriate?
- You don't need a library to implement State Machines. You can use patterns in each language.
- XState becomes more valuable when representing Statecharts.
- XState version 5 will be smaller. It will be explicit, small, and fast.
- See Twitter